In this article, you will learn to:
- How to Add Two Images
- How to Subtract Two images
- How to Multiply Two Images
Install and Import Necessary Libraries:
Install OpenCV using pip command.
pip install opencv-python
First, we import all necessary libraries like cv2, NumPy, and matplotlib, etc.
import cv2import numpy as npimport matplotlib.pyplot as plt
Read/load and Display Images
import cv2# load first imageimg1 = cv2.imread('lena.png')img1 = cv2.cvtColor(img1, cv2.COLOR_BGR2RGB)# load second imageimg2 = cv2.imread('OpenCV_logo.png')img2 = cv2.cvtColor(img2, cv2.COLOR_BGR2RGB)# resize the imagesimg1 = cv2.resize(img1,(500,500))img2 = cv2.resize(img2, (500,500))# display first imagecv2.imshow('image',img1)cv2.waitKey(0)cv2.destroyAllWindows()# display second imagecv2.imshow('image',img2)cv2.waitKey(0)cv2.destroyAllWindows()
Output:
![]() |
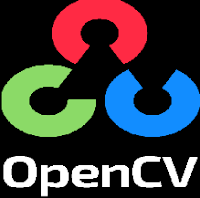
You may be interested in the below post to learn different ways to load images.
How to Add Two Images
Using cv2.add() Method
We can use OpenCV function cv2.add() to add two images, or simply we can use Numpy operation img1 + img2 to add the images.
cv2.add()
img1 = cv2.imread('lena.png')img2 = cv2.imread('OpenCV_logo_white.png')img = cv2.add(img1, img2) # 240+22 = 262 => 255cv2.imshow('image',img)cv2.waitKey(0)cv2.destroyAllWindows()
Output:
Notice that cv2.add() method adds two images of same size, i.e., same height and width. So we have resized the images to make them of same size.
We can add or subtract images of different sizes using Python-OpenCV without resizing them.
Using numpy operation img1 + img2
img1 = cv2.imread('lena.png')img2 = cv2.imread('OpenCV_logo_white.png')img = img1 + img2 # 240+22 = 262 % 256 => 6cv2.imshow('image',img)cv2.waitKey(0)cv2.destroyAllWindows()
Output:
Note the difference between the above two resultant images.
Image Blending
If you want to add two images with different weights, use cv2.addWeighted() function
img=α⋅img1+β⋅img2+γ
Here α and β are weights of first and second images and γ is a scalar quantity.
img1 = cv2.imread('lena.png')img2 = cv2.imread('OpenCV_logo_white.png')img = cv2.addWeighted(img1, 0.3, img2, 0.7, 0)cv2.imshow('image',img)cv2.waitKey(0)cv2.destroyAllWindows()
Output:
Add Two Images Vertically and Horizontally
We use cv2.vconcat([image1, image2]) and cv2.hconcat([image1, image2]) to add two images vertically and horizontally respectively.
import cv2import matplotlib.pyplot as plt#add images virticallyimg_v = cv2.vconcat([img1,img2])#add images horizontallyimg_h = cv2.hconcat([img1,img2])fig = plt.figure(figsize=(5, 5))rows, columns = 1, 2fig.add_subplot(rows, columns, 1)# showing vertical imageplt.imshow(img_v)plt.title("Virtical Add")fig.add_subplot(rows, columns, 2)# showing horizontal imageplt.imshow(img_h)plt.title("horizontal Add")
Output:
![]() |
Vertically and Horizontally added image output |
Note: Here, to display images we used plt.imshow() to show the pixel values.
import numpy as npvertical = np.vstack((img1, img2))horizontal = np.hstack((img1, img2))
How to Subtract Two Images
# subtract imagessub_cv = cv2.subtract(img1, img2)sub_np = np.subtract(img1,img2)sub_abs = cv2.absdiff(img1, img2)# define figure to display above three imagesfig = plt.figure(figsize=(5, 5))rows, columns = 2, 2# add first image to figurefig.add_subplot(rows, columns, 1)# showing imageplt.imshow(sub_cv)plt.axis('off')plt.title("cv2 image subtraction")# add second image to figurefig.add_subplot(rows, columns, 2)# showing imageplt.imshow(sub_np)plt.axis('off')plt.title("np image subtraction")# add third image to figurefig.add_subplot(rows, columns,# showing imageplt.imshow(sub_abs)plt.axis('off')plt.title("cv2 abs diff")
Output:
How to Multiply Two Images:
#multiply imagesmul_cv = cv2.multiply(img1, img2)mul_np = np.multiply(img1, img2)# sub_abs_np = np.abs(img1,img2)fig = plt.figure(figsize=(5, 5))rows, columns = 1, 2fig.add_subplot(rows, columns, 1)# showing imageplt.imshow(mul_cv)plt.axis('off')plt.title("cv2 image multiplication")fig.add_subplot(rows, columns, 2)# showing imageplt.imshow(mul_np)plt.axis('off')plt.title("np image multiplication")
Output:
![]() |
Image Multiplication |
Comments
Post a Comment